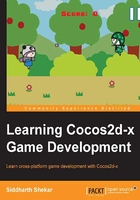
Displaying the background image
Now that we have a clean slate, let's start from the top by displaying the background image.
You might think that, "Wait a second. Are we going to be using a static image for the background? But the book says it will teach the parallax background." It is true that we are going to use a static background for the time being so that it is easy to visualize and see whether proportionately everything looks right on the screen. The image is just for reference; we will change the static image into a parallax layer later in the book.
First, we need to copy all the assets from the Resources
folder provided with the chapter into the Resources
folder of the project on the drive. The Resources
folder should be in the wp8Game
project folder along with the Classes
folder, as mentioned in Chapter 1, Getting Started. After importing the background image and other images into the Resources
folder, we go to the wp8Game project (not the component!) in the Solution Explorer pane in Visual Studio, click on the triangle beside it to open the folder structure, and look for the Resources
folder. We then right-click on the Resources
folder and navigate to Add | ExistingItem. Now, we navigate to the Resources
folder on the drive that we have copied all the resources of the game on, select the bookGame_Bg.png
file, and finally click on Add. We should now be able to see the file in the Solution Explorer pane, as follows:

Now, in the init()
function, we create a new variable called bg
of the type CCSprite
and position it as follows:
CCSprite* bg = CCSprite::create("bookGame_Bg.png"); bg->setPosition(ccp(visibleSize.width* 0.5, visibleSize.height * 0.5)); this->addChild(bg, -1);
Regular C++ users follow the practice of "if you create new, you must delete", which pertains to creating pointers. However, since CCSprite is an autorelease object of Cocos2d-x, this is not required in this case.
Now, if we build and run it, the background image will look as follows:

Note how the code is exactly the same as the initial default Cocos2d-x background that was displayed, except that I just put the depth as -1
so that the background object is behind all the other objects on the screen.
Congratulations! We have displayed the first object on the screen. Let's ship it and make some money. Well, not so soon. We still need our hero to make it a little more interesting. The player obviously won't pay a whole lot of money for a pretty picture to get displayed on the screen.
Next, we will import the hero into the game. So, we follow the same steps to import the bookGame_tinyBazooka.png
file into the project that we did for bg
. So, we go to the Resources tab in the Solution Explorer pane, right-click and select Add Existing item, select the PNG file, and click on Import.
As we will be accessing the hero sprite all the time, we will make it a global variable and declare it in the .h
file instead of the .cpp
file. So, we go to the .h
file and create a new CCSprite variable called hero
right below the area where it says public:
, as follows:
CCSprite* hero;
Now, below the position where we created bg
in the init()
function, we type the following:
hero = CCSprite::create("bookGame_tinyBazooka.png"); hero->setPosition(ccp(visibleSize.width * 0.25, visibleSize.height * 0.5)); addChild(hero, 5);
We now build and run the project; we should see the hero on the screen, as follows:

Unlike the background object that was placed at the center of the screen, the hero is placed at 0.25
or 1/4th the width of the screen and at half the height of the screen. I have placed it at a z depth of 5
so that it is above all the other objects on the screen. Now, if we add a new child between -1
and 5
, it would be placed between the background and the hero image.