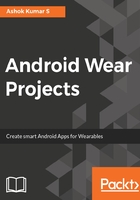
上QQ阅读APP看书,第一时间看更新
Working on activities and driving the project towards completion
In MainActivity.java, we will have control over the classes that we have worked on so far. Let's get started on implementing all the necessary code and methods.
When we extend the Wear project activity to WearableActivity, we shall include the following code in the manifest <application ></application> tag scope:
<uses-library android:name="com.google.android.wearable" android:required="false" />. When we declare android: required="false" any feature that Android has control on will be disabled. In the context of Wear applications, when you need to extend your activity with WearableActivity, we need to set it to false.
<uses-library android:name="com.google.android.wearable" android:required="false" />. When we declare android: required="false" any feature that Android has control on will be disabled. In the context of Wear applications, when you need to extend your activity with WearableActivity, we need to set it to false.
Now, in MainActivity.java, let's add the basic variable instances necessary for the project. Add the following code globally in the MainActivity.java class scope:
private static final String TAG = "MainActivity"; private static final int REQUEST_CODE = 1001; private RecyclerViewAdapter mAdapter; private List<Note> myDataSet = new ArrayList<>();
Next, write a method for configuring the UI component. Let's call this method ConfigureUI(). In this method, we shall handle the click event. When a user clicks on the RecyclerView list item, it should take the user to the delete activity screen:
private void configureUI() { WearableRecyclerView recyclerView = (WearableRecyclerView) findViewById(R.id.wearable_recycler_view); recyclerView.setHasFixedSize(true); LinearLayoutManager mLayoutManager = new LinearLayoutManager(this); recyclerView.setLayoutManager(mLayoutManager); mAdapter = new RecyclerViewAdapter(); mAdapter.setListNote(myDataSet); mAdapter.setListener(this); recyclerView.setAdapter(mAdapter); EditText editText = (EditText) findViewById(R.id.edit_text); editText.setOnEditorActionListener(new TextView.OnEditorActionListener() { @Override public boolean onEditorAction(TextView textView, int action, KeyEvent keyEvent) { if (action == EditorInfo.IME_ACTION_SEND) { String text = textView.getText().toString(); if (!TextUtils.isEmpty(text)) { //Todo add the on editText click handlers return true; } } return false; } }); }